My first ever icinga plugin
Icinga2 is a Monitoring Application. Its main purpose is to monitor services of specified hosts. You either see the errors on the Webpage or you receive a small mail with the error message, if configured. Icinga provides plugins like ping, ssh, diskspace and many more for those monitoring tasks, but you can create plugins as well. Plugins can be scripts (Shell, Python, Perl, Ruby, PHP, etc.) or compiled binaries (C, C++, Go). I really was curious about writing my own icinga2 plugin and here it is. Mine is completely written in Shell and solely tested on my QNAP TS-212 NAS. Don’t hesitate to give it a shot on your QNAP NAS and share the results with me.
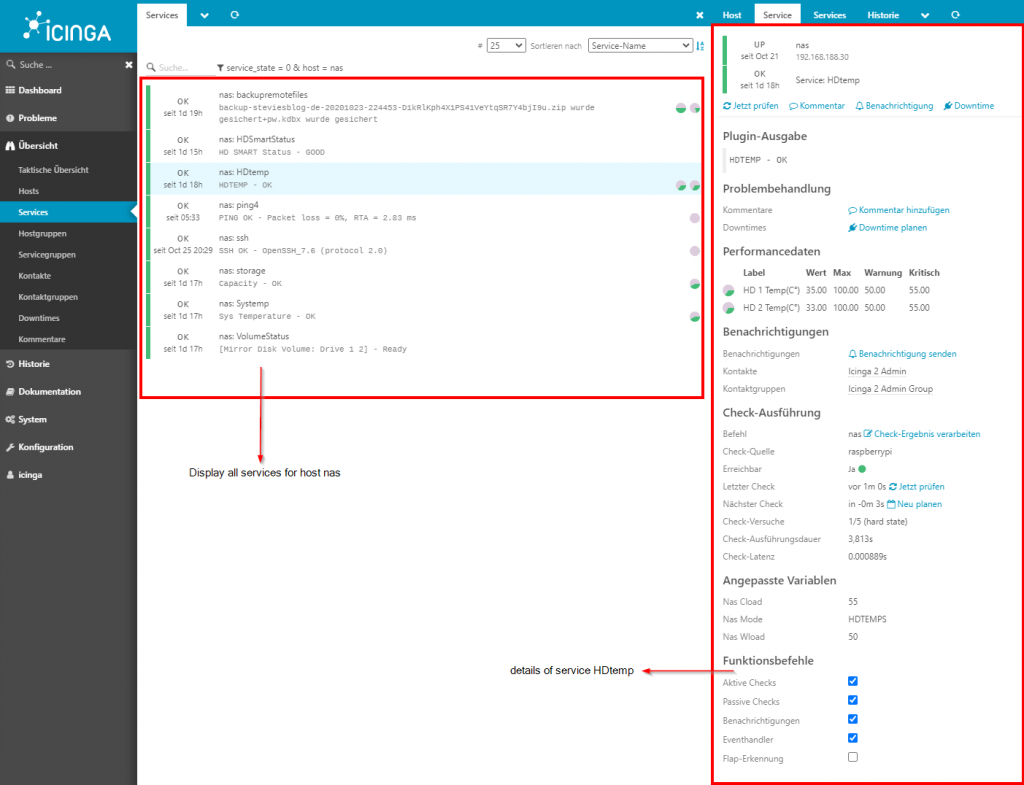
This is what my plugins output is on the webinterface. For now it contains a HDTemps, SysTemps, HDSmartStatus, HDCapacity and Volumestatus check. For each Hard drive, it will collect the temperature and the smart status. Were required you need to add a warning and critical threshold. To integrate this script to icinga you need to create a checkCommand object like this.
object CheckCommand "nas" { command = [ PluginDir + "/nas.sh"] arguments = { "-h" = { value = "$host.address$" description = "Hostname of the remote machine" } "-u" = { value = "$nas_username$" description = "Username for ssh Login" } "-w" = { value = "$nas_wload$" description = "Exit with WARNING Status if limit exceeds wload" } "-c" = { value = "$nas_cload$" description = "Exit with CRITICAL Status if limit exceeds wload" } "-m" = { value = "$nas_mode$" description = "mode for specific reports" } "-p" = { value = "$nas_port$" description = "ssh Port to connect to(default 22)" } } vars.nas_wload = 80 vars.nas_cload = 95 }
80 is the default thresold for a warning message whilst 95 is the same for a critical message.
Service Object
For simplicity sake i applied those services to each host where host.vars.nas_username is set. For example my capacity check looks like this:
apply Service "storage" { import "generic-service" check_command = "nas" vars.nas_mode = "CAPACITY" vars.nas_wload = 80 vars.nas_cload = 90 assign where host.vars.nas_username != "" }
With check_command = “nas” we call the previous defined CheckCommand Object with the same name. Nas_mode is always required to be set. You can see all available options by executing the script in the terminal.
pi@raspberrypi:/usr/lib/nagios/plugins $ ./nas.sh --help nas, version 2020.10.19 Usage: ./nas.sh [OPTIONS] Option GNU long option Meaning ------ --------------- ------- -q --help Show this message -v --version Print version information and exit -h --hostname set hostname/IP -u --username set username -p --port set Port default(22) -c --critical set critical value -w --warning set warning value -m --mode CAPACITY,BACKUP,VOLUME,SYSTEMP,HDTEMPS,HDDSTATUS
Find underneath my second example how i execute the hard drive temperature check:
apply Service "HDtemp" { import "generic-service" check_command = "nas" vars.nas_mode = "HDTEMPS" vars.nas_wload = 50 vars.nas_cload = 55 assign where host.vars.nas_username != "" }
Host Object
My nas host does have the variable nas_username =”admin” so the services are linked with the host object.
object Host "nas" { import "generic-host" address = "192.168.188.30" check_command = "hostalive" vars["os"] = "Linux" version = 1596033350.202298 zone = "raspberrypi" vars.nas_username = "admin" /* Define notification mail attributes for notification apply rules in `notifications.conf`. */ vars.notification["mail"] = { /* The UserGroup `icingaadmins` is defined in `users.conf`. */ groups = [ "icingaadmins" ] } }
To retrieve all those Informations about my QNAP nas i use the internal command getsysinfo, so make sure it is available on your nas as well.
OK, you came down to here and did not see any peace of my code :).
It is available on my github repository, so check it out and follow the link 🙂
https://github.com/stevieWoW/NAS-QNAP-ICINGA2
My Scripts requires ssh access to the nas system. To prevent a password prompt you need to add a private/public key relation between icinga2 and the nas. Create a ssh key on icinga2 with your nagios account and add that public key to the authorized hosts on your nas system.
I really appreciate any feedback so feel free to test it 🙂